반응형
https://programmers.co.kr/learn/courses/30/lessons/42584
코딩테스트 연습 - 주식가격
초 단위로 기록된 주식가격이 담긴 배열 prices가 매개변수로 주어질 때, 가격이 떨어지지 않은 기간은 몇 초인지를 return 하도록 solution 함수를 완성하세요. 제한사항 prices의 각 가격은 1 이상 10,00
programmers.co.kr
스택/큐 활용 X
import java.util.*;
class Solution {
public int[] solution(int[] prices) {
int[] answer = new int[prices.length];
for(int i=0; i<prices.length; i++){
for(int j=i+1; j<prices.length; j++){
if(prices[i] <= prices[j]){
answer[i]++;
}
else{
answer[i]++;
break;
}
}
}
return answer;
}
}
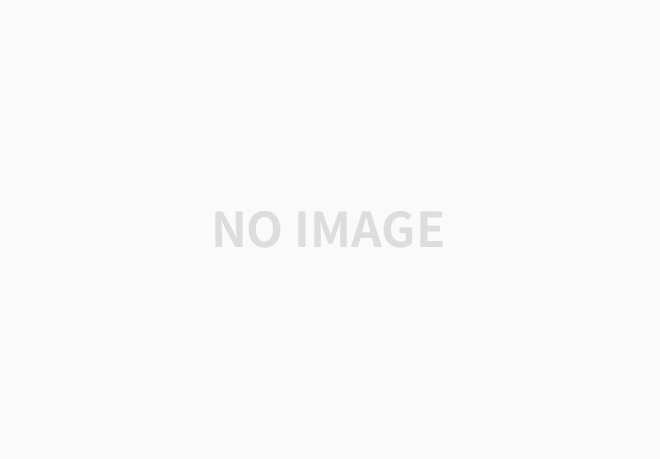
스택/큐 활용 O
import java.util.*;
class Solution {
public int[] solution(int[] prices) {
int[] answer = new int[prices.length];
Queue<Integer> que = new LinkedList<>();
for(int price : prices){
que.add(price);
}
int index=0;
while(!que.isEmpty()){
int cnt = 0;
//큐에서 하나 꺼낸다.
int queElement = que.poll();
//나머지 큐에 잇는거랑 비교한다.
for(int price : que){
//큐에 잇는값이 더 크면 카운트++
if(price >= queElement){
cnt++;
//큐에 잇는 값이 더 작으면 카운트++하고 포문을 나간다.
}else{
cnt++;
break;
}
}
answer[index] = cnt;
index++;
}
return answer;
}
}
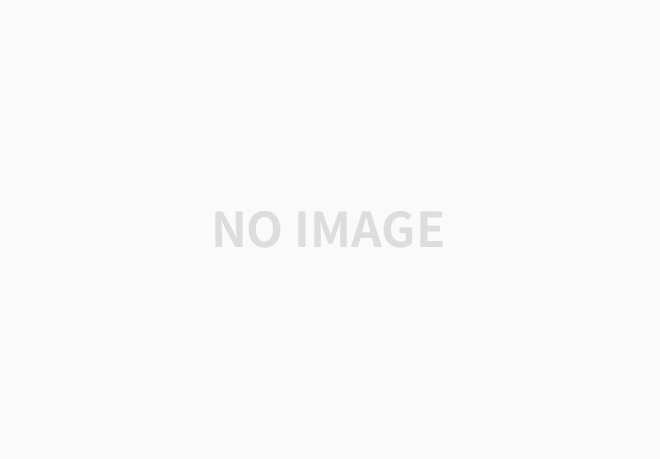
반응형
'코딩테스트 > 프로그래머스 2단계' 카테고리의 다른 글
프로그래머스 2단계 - 더 맵게(힙) (0) | 2021.09.13 |
---|---|
프로그래머스 2단계 - 프린터(스택/큐) (0) | 2021.09.09 |
프로그래머스 2단계 - 다리를 지나는 트럭(스택/큐) (0) | 2021.09.09 |
프로그래머스 2단계 - 기능개발(스택/큐) (0) | 2021.09.09 |
프로그래머스 2단계 - 위장(해쉬) (0) | 2021.09.08 |